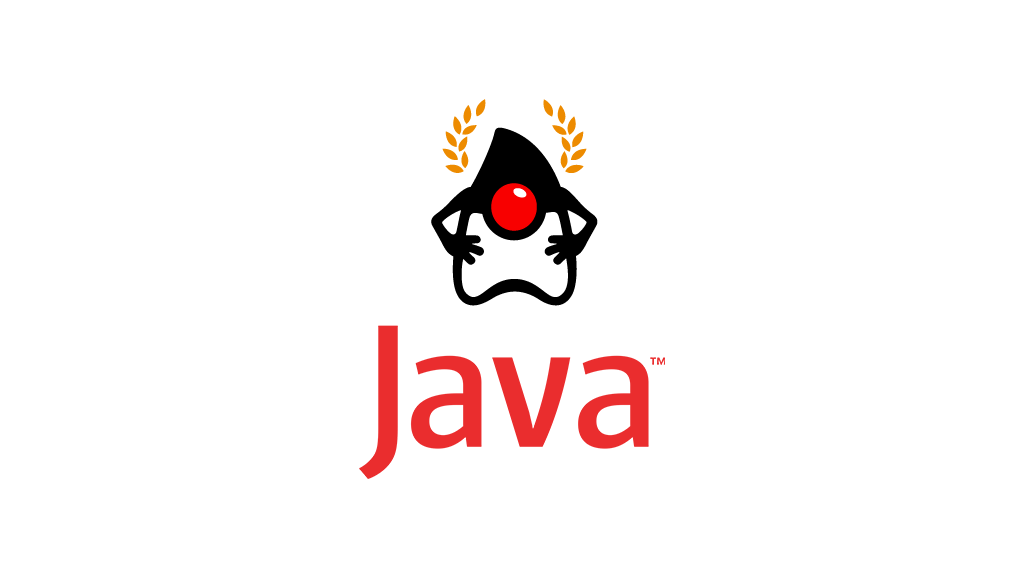
My goal here is to introduce some concepts of Java to have a better understanding of it.
Navigation
- Functional Interface
- Lambda Expression
- Mixed-type arithmetic
- Collection API
- Checked vs Unchecked Exceptions
- Java platform components
- Initialization Order
- Java String Pool
- equals() and hashCode()
1. Functional Interface
The functional interfaces (also known as Single Abstract Method Interface) have only one abstract method. As they have only one abstract method, Lambda expressions can define an implementation of these interfaces.
Since default methods have an implementation, they are not abstract.
Example:
public interface ActionListener extends EventListener { public void actionPerformed(ActionEvent e); }
Note that the annotation @FunctionalInterface allows a compiler to generate an error if the annotated interface does not satisfy the conditions.
2. Lambda Expression
Lambda Expressions can be used in place of Anonymous function in the code if it define a Functional Interface. These expressions can implement only one method.
A Lambda Expression is composed of a list of parameters and a body that can be a simple expression or a block of code.
( parameters ) -> expression;
( parameters ) -> { block of code ; }
You can omit the parenthesis if there is one parameter with a type that can be inferred.
Example with an Anonymous function for a Swing button and an ActionListener Interface:
button.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent event) { System.out.println("Button clicked"); } });
The same with a lambda expression:
button.addActionListener(event -> System.out.println("Button clicked"););
3. Mixed-type arithmetic
When an operation involves two data types, the smaller type is converted to the larger type.
Example : (int *double) is converted to (double*double)
The types byte, short and int in an arithmetic operations (+, −, *, /, %) are converted to int type before the arithmetic operation is performed.
short a = 2; short b = 3; // short c = a*b; This line does not compile. int c = a*b; //This line compile
If one of the operands is of type long, then all values will be converted to long.
4. Java Collection API
The diagram of the Java Collection API.
Set :
It is a collection that contains no duplicate elements. More formally, sets contain no pair of elements e1 and e2 such that e1.equals(e2), and at most one null element. As implied by its name, this interface models the mathematical set abstraction.
List :
It is an ordered collection (also known as a sequence). The user of this interface has precise control over where in the list each element is inserted. The user can access elements by their integer index (position in the list), and search for elements in the list.
Queue :
It is a collection designed for holding elements prior to processing. Queues provide additional insertion, extraction, and inspection operations. Each of these methods exists in two forms: one throws an exception if the operation fails, the other returns a special value (either null or false, depending on the operation). Queues typically, but do not necessarily, order elements in a FIFO manner.
5. Checked vs Unchecked Exceptions
There are two types of exceptions:
- Checked exceptions that are checked at compile time. They must either be handeld in the method with a try/catch block or they must be specified with the throws keyword.
- Unchecked exceptions that are not checked at compiled time.
6. Java platform components
JVM : Java Virtual Machine
Enables a computer to run Java programs as well as programs written in other languages that are also compiled to Java bytecode.
JDK : Java Development Kit
Includes tools useful for developing and testing programs written in the Java programming language and running on the Java platform.
JRE : Java Runtime Environment
Provides the minimum requirements for executing a Java application; it consists of the JVM, core classes, and supporting files.
JSE : Java Standard Edition
Defines a range of general-purpose APIs.
J2EE : Java Enterprise Edition ( rebranded as Jakarta EE)
Set of specifications, extending Java SE with specifications for enterprise features such as distributed computing and web services.
JSR : Java Specification Requests
The formal documents that describe proposed specifications and technologies for adding to the Java platform.
7. Initialization Order
Here is the execution order of the initialization fields and blocks, static and non-static with inheritance.
public class Example { static { step(1); } public static int step_2 = step(2); public int step_6 = step(6); public Example() { step(8); } { step(7); } // Just for demonstration purposes: public static int step(int step) { System.out.println("Step " + step); return step; } } public class ExampleSubclass extends Example { { step(9); } public static int step_3 = step(3); public int step_10 = step(10); static { step(4); } public ExampleSubclass() { step(11); } public static void main(String[] args) { step(5); new ExampleSubclass(); step(12); } }
Output :
Step 1
Step 2
Step 3
Step 4
Step 5
Step 6
Step 7
Step 8
Step 9
Step 10
Step 11
Step 12
Important points :
– Static blocks are always run before the object is created
– When you call a constructor of the subclass, it implicitly calls super();
before executing
– The code order matter between an initialization block and a field initializer
8. Java String Pool
String is immutable in Java and it implements the String interning concept.
9. equals() and hashCode()
The equals() method allows you to check whether an object is equal to another.
The default implementation of equals() :
public boolean equals(Object obj) { return (this == obj); }
The redefinition of the equals () method must respect several constraints:
to be continued …